Technical: OpenAPI Core Python Library with Some Hands-on Examples
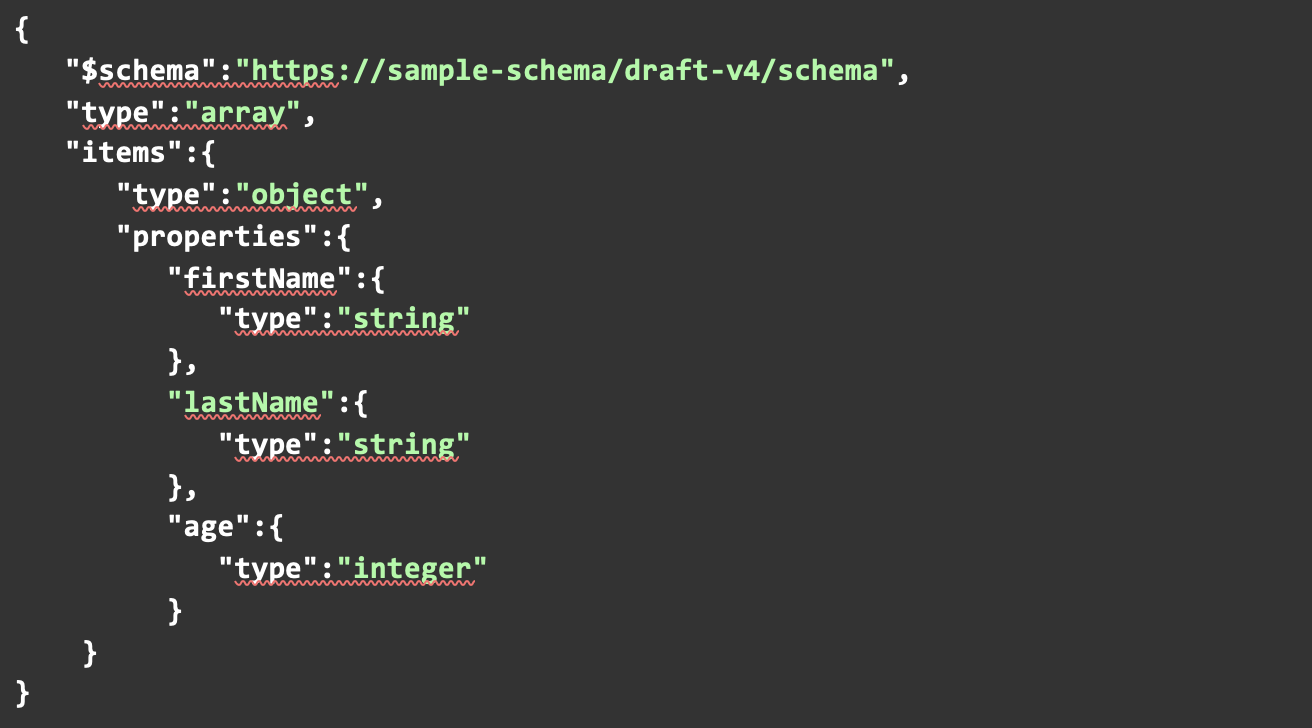
In this article, we will cover the OpenAPI core python library with some hands-on examples.
Open API core library:- Openapi-core is a Python library that adds client-side and server-side support for the OpenAPI Specification v3.
OpenAPI Specification:- OpenAPI(Swagger) is a specification for documenting REST API. Swagger scans the code and exposes the documentation on the given API. Any client can consume this URL (which comes as XML or JSON documents) and learn how to use REST web services: which HTTP methods to call on which URL, which input documents to send, which status code to expect, and so on. The OpenAPI specifications describe the API request (request body schema, query parameters, request headers) and define each field's desired data types and format in the response.
The intent of documenting the REST API is to provide ease to the clients where they can quickly try out the web services without any manual assistance.
An example of OpenAPI Specification:
Explanation:
$schema: The $schema keyword states that this schema is written according to the draft v4 specification.
type: The type keyword defines the datatype constraint on the JSON data. Here type: array defines the data should be an array (list of items).
items: items represents the properties of the item of an array.
properties: properties defines the properties of an object's elements. The object here should contain firstName, lastName, and age elements.
OpenAPI core library features-
- Validation of requests and responses: Validate the request and response against the given specification. Throw an error if any mismatch is found.
- Schema casting and unmarshalling: Convert JSON Schema into Python data types.
- Media type and parameters deserialization
- Custom deserializers and formats: Create your own deserializers to deserialize the particular data.
- Integration with libraries and frameworks: Can be integrated with Django
This article will focus on the validation of requests and responses against OpenAPI specifications.
Request Validation using the openapi-core library:
A request is made by the client to the server to access a resource of a server. A request may contain the following elements -
- Request line (It contains information about the requests method, the path component of the URL for the request, and the HTTP version number).
- Request headers
- Request payload (if require)
Using request validation, we can validate the following elements of the request -
- Query: Query is used for filtering the response data. It is specified at the end of the URL after the question mark (?). For example, https://{url}?id=1. Here id=1 is a query.
- Headers are used to pass additional information with an HTTP request. The most common headers are- Authorization (used for authorization for accessing the resource), Host (The Host request-header specifies the host and port number of the server to which the request is being sent), Content-Type (MimeType of request payload), Content-Length (length of payload data).
- Cookie
Creating the spec:- We need to create a specification object using openapi.json. The openapi.json file can be created with the swagger tool(https://swagger.io/). A sample openapi.json file is uploaded here.(FILE Link)
The spec structure is
servers
paths
{endpoint}
{request_method}
requestBody
parameters
responses
{status_code}
content
{response_body_mimetype}
Creating an OpenAPIRequest object:- For validating the request, we need to create an object of OpenAPIRequest class.
Validate the request: Now that we have Spec and OpenAPIRequest objects, we can validate our request against the given openapi.json (OpenAPI specification) -
Response Validation using the openapi-core library: The response from the server can contain the following elements -
- Status-Code: Status-Code indicates the result of the request. The status codes are defined under section 10 of RFC 2616.
- Data: The response content or response body.
- Response headers: meta-information of response
For schema validation of response, we need to follow the below steps -
Creating the spec:- We need to create the spec similarly as mentioned above. Generally, spec contains the information about requests and responses. So same openapi.json file will be used to create the Spec class object.
Creating an OpenAPIResponse object:
Validate the response: Validate the response by passing the above response object in ResponseValidator class object
Why we need to pass response and request both in response_validator.validate method:
The response (OpenAPIResponse class object) contains information about the response body, response status code, and response-body mimetype. To reach the correct response schema, we need an endpoint and request_method(as shown in the spec structure hierarchy). The endpoint and request method information is carried by request (OpenAPIRequest class object). So, we need to pass response and request both in response_validator.validate method.
We have learned about a powerful tool that can validate the request and response against given OpenAPI specifications, no matter how long our response is. The openapi-core library is normally used in Test Automation, where we need to validate the API response’s attribute properties.
Feel like you could use a hand?
See what’s possible and give your teams the ability to create positive change.
Contact NowElevate your tech savvy! Warning: May cause increased knowledge.
Exclusive technology and development insights, tips, and podcasts await.